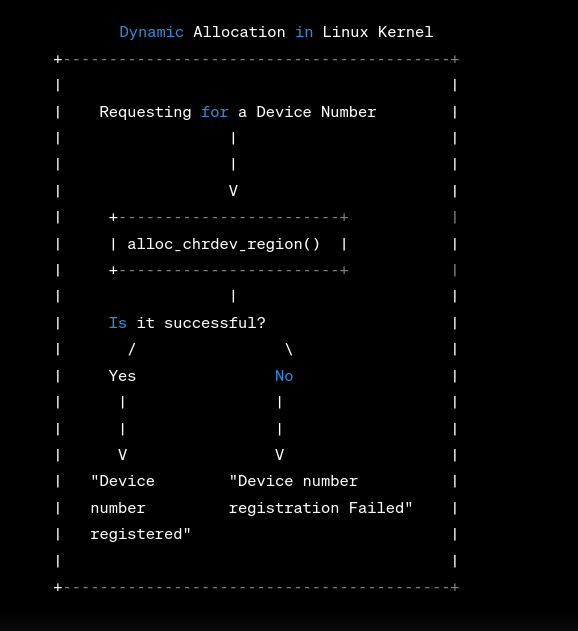
Hello tech enthusiasts! 🚀
Today, we are taking a deep dive into the world of Linux Kernel Modules and one of its star players, Dynamic Allocation. If you’ve ever been curious about how Linux manages device numbers or are looking to write your own device driver, this post is for you.
🎯 Setting the Stage: What are Device Numbers?
Device numbers, consisting of a major and minor number, are pivotal in the Linux Kernel’s device-driver linkage. The major number identifies the driver associated with the device, while the minor number differentiates between various devices the driver may control. Traditionally, these numbers were statically allocated, which can be restrictive and clash-prone.
Enter: Dynamic Allocation.
🌟 Dynamic Allocation: The Hero We Needed
Dynamic Allocation allows the Linux Kernel to assign major numbers dynamically, ensuring no clashes and optimal management. Let’s think of it as a hotel concierge giving you the next best available room without you having to request a specific one.
From our class notes, we learn about a nifty function: alloc_chrdev_region()
. This is the magic spell that requests the kernel for a range of device numbers, allowing flexibility in device creation.
💻 A Sneak Peek into Code
Here’s a basic example where we define some parameters like minor numbers, count, and device name:
int base_minor = 0;
char *device_name = "mychardev";
int count = 1;
dev_t devicenumber;
These parameters can even be overridden when inserting the module using the insmod
command, providing immense flexibility:
module_param(base_minor, int, 0);
module_param(count, int, 0);
module_param(device_name, charp, 0);
The real action starts in the test_hello_init
function:
if (!alloc_chrdev_region(&devicenumber, base_minor, count, device_name)) {
printk("Device number registered\n");
printk("Major number received:%d\n", MAJOR(devicenumber));
}
else {
printk("Device number registration Failed\n");
}
Here, we’re trying to allocate a character device number. If all goes well, we celebrate by printing the allocated major number. But if it fails, well, we get a clear log message about the failure.
🔄 A Goodbye is Just a New Beginning
When done with the device, it’s good manners (and great coding) to free up the resources. The unregister_chrdev_region
function ensures we don’t hog the device numbers:
unregister_chrdev_region(devicenumber, count);
🧐 Wrapping Up: What’s the Big Deal?
Why all this fuss about dynamic allocation, you ask?
- Flexibility: By allowing kernel-driven allocations, we reduce manual overhead.
- Optimal Management: Minimized risk of conflicts with other device numbers.
- Scalability: Especially important in systems with a large number of dynamically created devices.
The Kernel world is vast and intriguing. Dynamic allocation is just one of its many facets. But as we’ve seen, it plays a pivotal role in simplifying device-driver interactions, making the life of a kernel developer that much easier.
If you’ve got any fascinating insights, experiences, or questions on this topic, drop them in the comments. Let’s keep the tech conversation buzzing! 🐝
Happy coding! 💻