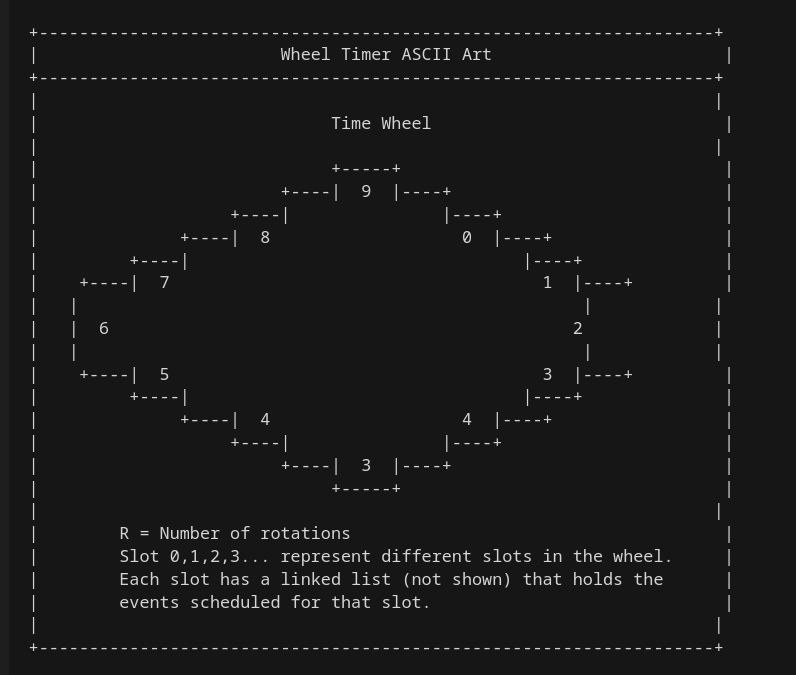
Wheel Timers: The Tick-Tock of Event Scheduling in Programming
ย
Introduction ๐
Welcome to a deep dive into one of the most fascinating data structures out thereโthe Wheel Timer! If you’re in the business of efficiently managing timing events, this structure is a must-know. This article will walk you through the basic design, underlying data structure, and the C implementation of Wheel Timers.
๐ GitHub Repository
ย
Basic Design of Wheel Timer ๐ก
A Wheel Timer is essentially a Linux timer fused with a wheel data structure. Think of it as a wall clock with multiple slots, each representing a unit of time. Though a traditional clock has 60 slots, we’ll use a simplified version with 10 slots for this demonstration.
ย
Linked List in Slots ๐๏ธ
Each slot in the Wheel Timer carries a linked list of events. When the “needle” of the timer (analogous to the second hand of a clock) hits a particular slot, all events in the linked list get fired.
ย
Complete Rotations ๐
The timer maintains a variable ‘R’ to track the number of complete rotations made. This helps in rescheduling and repeated triggering of events.
ย
Event Triggering & Scheduling ๐
Events are essentially function pointers. When the timer’s needle hits a slot, the corresponding events are triggered sequentially. Events can also be set to repeat at intervals, making it highly flexible for a range of applications.
ย
Dynamic Event Scheduling ๐๏ธ
To schedule an event, you’d calculate the slot where the new event should reside. For instance, if the timer is currently at Slot 2 and you want the event to trigger after 3 seconds, you’d place it in Slot 5.
ย
Rescheduling Events ๐
Events can be rescheduled after they’re triggered. This is done by calculating Current Slot + Time Interval
.
ย
C Implementation of Wheel Timer ๐ ๏ธ
The core of the Wheel Timer is represented in C as a struct with six crucial members.
typedef struct _wheel_timer_t {
int current_clock_tic;
int clock_tic_interval;
int wheel_size;
int current_cycle_no;
_pthread_t *wheel_thread;
pthread_mutex_t wheel_timer_mutex;
ll_t *slots[0];
} wheel_timer_t;
ย
Initialization ๐๏ธ
The Wheel Timer is initialized by calling init_wheel_timer
, which takes two argumentsโthe number of slots and the clock tick interval.
wheel_timer_t* init_wheel_timer(int wheel_size, int clock_tic_interval)
ย
Running the Wheel Timer ๐โโ๏ธ
The timer runs on a separate thread, started by the start_wheel_timer
function. This function sets up a new POSIX thread to begin executing wheel_fn
, the function containing the timer’s core logic.
ย
Why This Matters ๐ฏ
Understanding the Wheel Timer can offer unparalleled efficiency in tasks that require time-based event management. Itโs commonly used in networking, real-time gaming, and many other time-sensitive applications.
ย
GitHub Repository ๐ฆ
All the code discussed in this blog post, including the C implementation of the Wheel Timer, can be found in the GitHub repository linked below.
๐ https://github.com/ANSANJAY/WheelTimer
ย
Conclusion ๐
Wheel Timers are extremely efficient for certain types of problems, especially those that require precise time management. If you’re a developer looking to expand your toolkit, understanding how to implement and use a Wheel Timer can be invaluable.
๐ Interested in More?: If you’re looking to dive deeper, the GitHub repository contains all the source code and additional resources to get you started.
ย